A common question I see is what is the best “pro” language for students to start with? There is no one right answer for this, because it depends on the kind of student and what your final objective is. I have some experience teaching a number of languages and know more of them, so I am going to offer some thoughts about starting with different languages that I hope help a teacher decide. When I say a “pro” language, I am speaking about something that is actually used for professional applications. That means obviously not Scratch, but also not something like Processing. These are both great languages to practice with before you learn one of the languages below, but as far as I know no one is creating any commercial applications in Processing or Scratch. I won’t address every possible language, because some of them (like Lisp) I just don’t know much about. I am going to do a series of posts starting with this same paragraph.
The first language I’ll address is Python, because it’s a popular learning language. As a beginning language Python has an enormous amount to recommend it. First of all it’s the most accessible language that I know of that’s actually used for professional code. You can get students doing something very quickly.
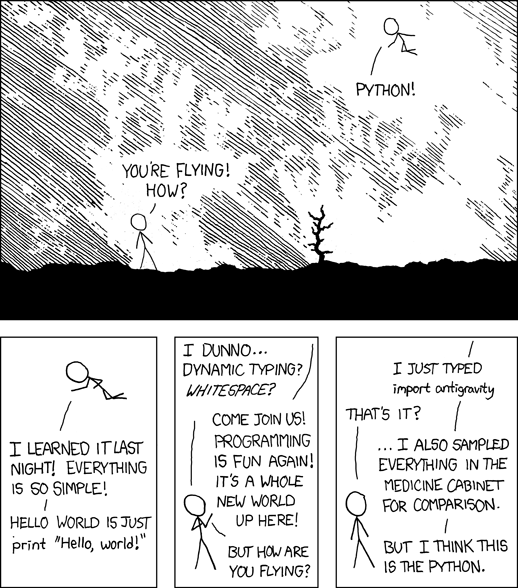
It also has an enormous number of modules that users can download and include in their programs to get them doing some fairly advanced stuff fairly quickly. The creation of blocks through indenting is different from how most languages do it. But even when your blocks are enclosed in curly brackets as with C-derived languages, indenting them is the expected style. So it’s not so hard to go from:
def addNumbers(a, b):
“Add some numbers”
c = a + b
return c
to:
int addNumbers(int a, int b)
{
//Add some numbers
int c = a + b;
return c;
}
And yet I no longer start my students with Python. Why not? Two reasons:
Notice the main difference about the two functions above, not counting punctuation? Yes, it’s the data type ‘int.’ To me, when students get to this point I want them to be thinking about data types. We expect the function above to be called as addNumbers(20, 50). But in Python, what’s going to stop a person from typing addNumbers(“Hello “, “world”)? If you do that, you’ll get a string back instead of an integer. But if you look at the function you wouldn’t expect that outcome, and you wouldn’t know that if you were passing that answer to a different function that was not capable of handling strings. Worse, if instead you called addNumbers(“20”, “50”) — which could easily happen if the numbers are in variables and you’re careless about how you handle user input — then you’d get back a string that looked like a number (though the wrong number, “2050”).
The second reason is that Python is an interpreted language, not a compiled one. This means that you run a Python program straight from the source code. Python will just run until something doesn’t work, then it will stop with an error. This means students will not be exposed to the crucial difference between two kinds of errors: a compile-time or syntax error and a runtime error or exception. Compiled languages like C have to first be turned into code that the machine can understand. If you attempted to call the addNumbers function as addNumbers(“20”, “50”) in a compiled language like C, Java or C++ the program would probably* not even compile, and you’d be informed that you tried to use strings where the program expected integers.
Say, however, that you have the user typing in the numbers in a compiled language. You could use code to tell a compiler to turn the user’s input into integers, so “50” becomes 50. The program will compile, because it will work if the user types in the right input. However, if the user types things that can’t be turned into numbers, like “Hello”, “World”, (or a decimal or a very big number that doesn’t fit in the integer data type) the program will probably* stop while running and return an exception. This is a vey different type of problem, and it helps teach students that even if you program does work, you have to prepare for getting the wrong kind of input that can make your program break.
However, you may disagree with me about the importance of these factors. If you just want your students to learn some basic programmatic thinking, Python may be the best starting place. You may feel that dealing with compiling and data types will turn off students who are lukewarm to coding.You might feel that Python is a good “bridge” between something like Processing and compiled, strictly typed languages like Java, then this also might be the best choice for you. And if Python is all you want your students to be able to do then of course teach them Python. After all, I know professional programmers for whom Python is their bread and butter.
If you do decide to teach your kids Python, the Python Software Foundation has a great tutorial here. I would recommend using this as a source rather than a direct teaching tool, unless you have very self-motivated students. Al Sweigart also has a great series of Python books here that I have used to good effect.
Next up I’ll be discussing starting students with Javascript.
*I say “probably” because it depends on the language and compiler; some compilers might just turn them into the number 0; and if it’s C maybe it would just use the pointers to the strings or something so you’d get an answer like -9832386 or something, because C is really hard as I’ll explain in a later post.